자바 클래스 기본개념 및 기본예제
https://developernew.tistory.com/21
JAVA 코딩 객체지향프로그래밍 OOP, 클래스 Class, 함수, 메소드
객체 지향 프로그래밍(OOP: Object Oriented Programming) 객체 중심의 프로그래밍 방식 객체끼리의 상호 작용을 통하여 프로그램을 작성하는 방식 부품화 캡슐화 == 클래스 속성과 기능을 하나의 캡슐처
developernew.tistory.com
JAVA oop class : static
: 객체 생성과 상관없이 사용되는 멤버
클래스명을 통해 접근하면 된다
-> 아무리 클래스 내부더라도 클래스명을 통해 접근하는 것을 권장한다
static 멤버변수
: 모든 객체가 일괄적으로 처리되어야 할 데이터를 저장하는 멤버변수 공간
모든 객체를 통틀어 단 하나의 공간만 만들어진다
모든 객체가 하나의 공간을 참조하게 된다 ; static 영역
프로그램이 실행할 때 공간이 만들어지고 프로그램이 끝날 때 공간이 소멸된다
; 객체 생성 이전에 먼저 만들어지는 공간
// Test class
public class Test {
int no; // 객체 번호
static int su; // 객체 수
public Test(int no, int su) {
this.no = no;
Test.su = su;
}
public static void setSu(int su) {
Test.su=su;
}
public int getSu() {
return su;
}
public void disp() {
System.out.println("객체 번호 : " + no + "\t객체 수 : " + su);
}
public void addSu() {
su++;
}
}
// Main class
public class Main {
public static void main(String[] args) {
// Math.random();
// Thread.sleep(0);
// System.currentTimeMillis();
Test.su = 10;
System.out.println(Test.su);
Test t1 = new Test(1, 1);
Test t2 = new Test(2, 3);
Test t3 = new Test(2, 3);
t1.disp();
t2.disp();
t3.disp();
}
static 메소드
: 클래스의 기능과는 연관이 있지만 객체 생성과는 상관 없는 기능을 만들 때 사용하는 메소드
this는 사용할 수 없다
static 멤버만 사용 가능하다
Exercise 1
이름, 알고리즘, 인공지능, 이산수학, 총점, 평균 - 일반 변수
알고리즘_총계, 인공지능_총계, 이산수학_총계 - static 변수
총점_총계, 평균_총계, 학생수_카운트 - static 변수
showDate - 멤버변수 내용 출력
showTotal - 멤버변수들의 총계 출력, static 메소드
(출력 결과)
이름 알고리즘 인공지능 이산수학 총점 평균
------------------------------------------------
장동건 100 100 100 300 100
원빈 98 78 100 276 92
김태희 28 78 30 136 45.3333
한가인 100 92 82 274 91.3333
================================================
총계 326 348 312 986 82.1667
// Public class
public class Score {
/* step1 변수 선언
이름, 알고리즘, 인공지능, 이산수학, 총점, 평균 - 일반 변수
알고리즘_총계, 인공지능_총계, 이산수학_총계 - static 변수
총점_총계, 평균_총계, 학생수_카운트 - static 변수
*/
private String name;
private int ag, ai, dm, tot;
private double avg;
private static int ag_tot, ai_tot, dm_tot, tot_tot, su;
private static double avg_tot;
// 생성자
public Score(String name, int ag, int ai, int dm) {
su++;
this.setAg(ag);
this.setAi(ai);
this.setDm(dm);
this.name=name;
}
// setter
public void setName(String name) {
this.name=name;
}
public void setAg (int ag) {
if(ag < 0 || ag > 100) {
System.out.println("잘못된 점수 입력");
return;
}
setAg_tot(ag);
this.ag=ag;
setTot();
setAvg();
}
public void setAi (int ai) {
if(ai < 0 || ai > 100) {
System.out.println("잘못된 점수 입력");
return;
}
setAi_tot(ai);
this.ai=ai;
setTot();
setAvg();
}
public void setDm (int dm) {
if(dm < 0 || dm > 100) {
System.out.println("잘못된 점수 입력");
return;
}
setDm_tot(dm);
this.dm=dm;
setTot();
setAvg();
}
private void setTot () {
this.tot=ai+ag+dm;
}
private void setAvg () {
this.avg=tot/3.0;
}
private void setAg_tot (int ag) {
Score.ag_tot-=this.ag;
Score.ag_tot+=ag;
setTot_tot();
setAvg_tot();
}
private void setAi_tot (int ai) {
Score.ai_tot-=this.ai;
Score.ai_tot+=ai;
setTot_tot();
setAvg_tot(); }
private void setDm_tot (int dm) {
Score.dm_tot-=this.dm;
Score.dm_tot+=dm;
setTot_tot();
setAvg_tot();
}
public void setSu() {
this.su=su;
}
public static void setTot_tot() {
Score.tot_tot = ag_tot + ai_tot + dm_tot;
}
public static void setAvg_tot() {
Score.avg_tot = tot_tot / (double)su / 3.0;
}
// getter
private String getName() {
return name;
}
private int getAg() {
return ag;
}
private int getAi() {
return ai;
}
private int getDm() {
return dm;
}
private int getTot() {
return tot;
}
private double getAvg() {
return avg;
}
private int getAg_tot() {
return ag_tot;
}
private int getAi_tot() {
return ai_tot;
}
private int getDm_tot() {
return dm_tot;
}
private int getTot_tot() {
return tot_tot;
}
private double getAvg_tot() {
return avg_tot;
}
public static int getSu() {
return su;
}
// user method
public void showDate () {
String print = String.format("%-8s %-10d %-10d %-10d %-6d %-10.4f", name,ag,ai,dm,tot,avg);
System.out.println(print);
}
public static void showTotal () {
String print = String.format("%-8s %-10d %-10d %-10d %-6d %-10.4f", "총계", ag_tot, ai_tot, dm_tot, tot_tot, avg_tot);
System.out.println(print);
}
// Main class
public class Main {
public static void main (String[] args) {
Score s1 = new Score("장동건", 100, 100, 100);
Score s2 = new Score("원빈", 98, 78, 100);
Score s3 = new Score("김태희", 28, 78, 30);
Score s4 = new Score("한가인", 100, 92, 82);
System.out.println(String.format("%-8s %-10s %-10s %-10s %-6s %-10s\n", "이름","알고리즘","인공지능","이산수학","총점","평균"));
// -(왼쪽정렬) 8(공백자릿수)
s1.showDate();
s2.showDate();
s3.showDate();
s4.showDate();
System.out.println("===================================================================");
Score.showTotal();
System.out.println();
System.out.println();
// 변수 중 s1의 ag점수와 s2의 dm점수를 변경해봄
s1.setAg(50);
s2.setDm(50);
System.out.println(String.format("%-8s %-10s %-10s %-10s %-6s %-10s\n", "이름","알고리즘","인공지능","이산수학","총점","평균"));
s1.showDate();
s2.showDate();
s3.showDate();
s4.showDate();
System.out.println("===================================================================");
Score.showTotal();
}
출력화면
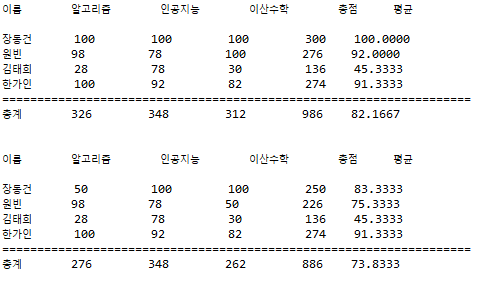
'Backend > JAVA' 카테고리의 다른 글
JAVA 코딩 객체지향프로그래밍 OOP, 배열 클래스 Array Class (0) | 2023.02.22 |
---|---|
JAVA 코딩 객체지향프로그래밍 OOP, Class 중첩클래스 - 일반중첩클래스, static중첩클래스, 지역중첩클래스, 익명중첩클래스 (0) | 2023.02.21 |
JAVA 코딩 객체지향프로그래밍 OOP, 클래스 Class, 함수, 메소드 (0) | 2023.02.16 |
JAVA 코딩 제어문 - 반복문 : while, for, do ~ while문 (0) | 2023.02.06 |
JAVA 코딩 제어문 - 선택문 : switch ~ case문 (0) | 2023.02.03 |