상속
: 부모클래스의 기능을 자식클래스가 물려받는 것
부모클래스에서 작성한 기능을 재사용하기 위해
class 클래스명 extends 부모클래스명 {
...
}
ex.
public class inheritance {
public static void main(String[] args) {
Student st = new Student();
st.breath();
st.learn();
Teacher t = new Teacher();
t.eat();
t.teach();
}
}
class Person {
void breath() {
System.out.println("숨쉬기");
}
void eat() {
System.out.println("밥먹기");
}
void say () {
System.out.println("말하기");
}
}
class Student extends Person {
void learn () {
System.out.println("배우기");
}
}
class Teacher extends Person {
void teach () {
System.out.println("가르치기");
}
}
//속 시에 생성자는 상속되지 않는다
상속 시 주의사항
JAVA에서는 다중상속을 지원하지 않는다
클래스 앞 final은 다른 클래스가 상속 불가
오버라이딩
: 자식클래스에서 부모클래스로부터 받아 온 메서드를 재정의하는 것
자식에 맞는 기능으로 맞춰 동작하기 위해
@
오버로딩 : 메서드 중복정의
VS
오버라이딩 : 메서드 재정의
상속관계에 있는 자녀클래스가 부모클래스에 있는 메서드를 재정의하는 것
ex.
public class Overriding {
public static void main(String[] args) {
Leader ld = new Leader();
ld.learn();
ld.lead();
ld.say();
}
}
class Student2 {
void learn () {
System.out.println("배우기");
}
void eat () {
System.out.println("밥먹기");
}
void say () {
System.out.println("선생님 안녕하세요");
}
}
class Leader extends Student2 {
void lead () {
System.out.println("반장으로 솔선수범하겠습니다.");
}
void say () {
System.out.println("선생님께 인사!");
super.say();
}
}
출력화면
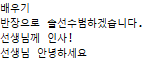
super( )
: 부모클래스의 생성자 호출
무조건 자식 클래스의 생성자 첫 줄에서 이뤄짐
작성하지 않을 시 , 컴파일러가 자동 호출
public class Object {
public static void main(String[] args) {
Aclass a1 = new Aclass();
Aclass a2 = new Aclass();
System.out.println(a1.toString());
System.out.println(a1);
// 객체정보의 문자열 출력
System.out.println(a1.equals(a1));
// 두 객체가 동일한가를 비교
System.out.println(a1.getClass());
// 객체의 클래스정보를 가져옴
}
}
class Aclass {
public String toString () {
return "Bclass 객체";
}
public boolean equals (Object obj) {
return true;
}
}
출력화면
JAVA Eclipse 01 프로그램, 프로그래밍, 기계어, JAVA
https://developernew.tistory.com/63
JAVA Eclipse 02 자바 출력메서드와 입력메서드
https://developernew.tistory.com/71
JAVA Eclipse 03 변수, 자료형, 형변환, 변수의 상수화
https://developernew.tistory.com/74
JAVA Eclipse 04 연산자 정의, 연산자 종류, 연산자 우선순위
https://developernew.tistory.com/78
JAVA Eclipse 05 논리연산자, 비트연산자
https://developernew.tistory.com/80
JAVA Eclipse 06 기타연산자 - 삼항연산자, 대입연산자, 복합대입연산자, instanceof
https://developernew.tistory.com/84
JAVA Eclipse 07 제어문 : 조건문
https://developernew.tistory.com/88
JAVA Eclipse 08 제어문 : 조건문 switch + Random 클래스
https://developernew.tistory.com/93
JAVA Eclipse 09 제어문 : 반복문 for
https://developernew.tistory.com/102
JAVA Eclipse 10 제어문 : 반복문 while, do-while
https://developernew.tistory.com/103
JAVA Eclipse 11 제어문 : 반복문의 break, continue
https://developernew.tistory.com/106
JAVA Eclipse 12 배열 : 배열의 개념 및 사용
https://developernew.tistory.com/107
JAVA Eclipse 13 배열 : 예제풀이, 로또번호 생성기
https://developernew.tistory.com/115
JAVA Eclipse 14 배열 : 다차원배열
https://developernew.tistory.com/117
JAVA Eclipse 15 배열의 복제, for ~ each문
https://developernew.tistory.com/120
JAVA Eclipse 16 카페 주문 시스템(키오스크) 배열과 제어문(반복문, 조건문)으로 풀기
https://developernew.tistory.com/124
JAVA Eclipse 17 method 메서드(메소드)
https://developernew.tistory.com/126
JAVA Eclipse 18 메서드(메소드) 오버로딩
https://developernew.tistory.com/133
JAVA Eclipse 19 객체지향 언어
https://developernew.tistory.com/135
JAVA Eclipse 20 클래스와 객체
https://developernew.tistory.com/155
JAVA Eclipse 21 인스턴스 변수와 클래스 변수
https://developernew.tistory.com/156
JAVA Eclipse 22 객체 타입 배열
https://developernew.tistory.com/157
JAVA Eclipse 23 클래스 생성자
https://developernew.tistory.com/158
'Backend > JAVA2 멘토시리즈' 카테고리의 다른 글
JAVA Eclipse 26 추상메서드와 추상클래스 (0) | 2023.05.11 |
---|---|
JAVA Eclipse 25 상속과 다형성 - 다형성 개념과 다형성 관련 실습 (1) | 2023.05.11 |
JAVA Eclipse 23 클래스 생성자 (0) | 2023.05.09 |
JAVA Eclipse 22 객체 타입 배열 (0) | 2023.05.08 |
JAVA Eclipse 21 인스턴스 변수와 클래스 변수 (0) | 2023.05.07 |